일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- TextMeshPro
- 직장인자기계발
- Unity Editor
- adfit
- 직장인공부
- base64
- 패스트캠퍼스후기
- Dots
- 환급챌린지
- Framework
- AES
- 가이드
- C#
- job
- DotsTween
- 샘플
- 커스텀 패키지
- 최적화
- 오공완
- 프레임워크
- unity
- Job 시스템
- sha
- 2D Camera
- 패스트캠퍼스
- RSA
- 암호화
- ui
- Custom Package
- Tween
Archives
- Today
- Total
EveryDay.DevUp
[Unity] Update() vs Job, Burst 성능 비교 본문
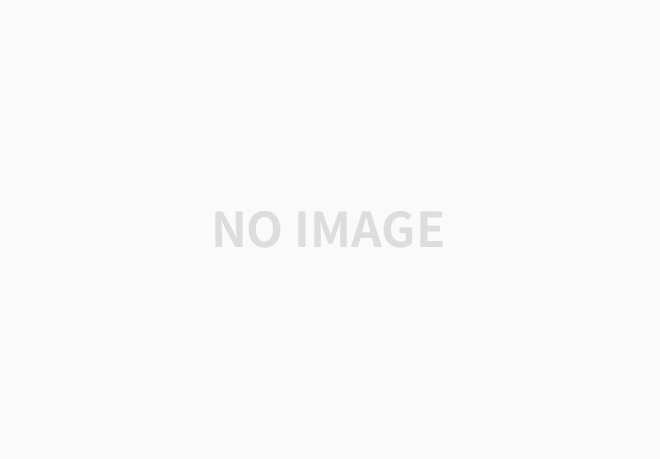
ITween은 MonoBehaviour의 Update() 를 통해 Tween이 동작하는데, DotsTween도 동일하게 Update() 를 사용하지만 Job, Burst를 구현하여 동작하는 방식을 사용한다.
ITween에서 동작하는 방식이 Job,Burst 보다 성능적으로 좋다면 Job, Burst를 사용할 의미가 없기 때문에 두 방식의 성능 차이를 비교하고자 한다.
Update에서 1000000개의 float 데이터를 변경할 때의 성능을 비교함으로써 Job, Burst의 효과를 알아보고자 한다.
public class JobMain : MonoBehaviour
{
int count = 1000000;
float[] testValues;
private void Start()
{
testValues = new float[count];
for( int i = 0; i < count; i++ )
{
testValues[i] = 0;
}
}
void Update()
{
for( int i = 0; i < count; i++ )
{
testValues[i] += math.sin( Time.deltaTime );
}
Debug.LogWarning( "0 Index : " + testValues[0] );
}
}
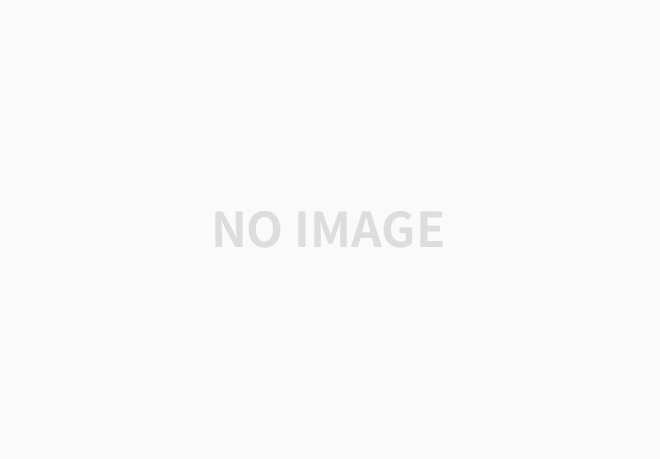
Update() 만을 사용했을 때, 12 fps 정도의 성능을 보인다.
public struct JobTest : IJobParallelFor
{
public float deltaTime;
public NativeArray<float> result;
public void Execute(int index)
{
result[index] += math.sin( deltaTime );
}
}
public class JobMain : MonoBehaviour
{
int count = 1000000;
float[] testValues;
private void Start()
{
testValues = new float[count];
for( int i = 0; i < count; i++ )
{
testValues[i] = 0;
}
}
void Update()
{
NativeArray<float> results = new NativeArray<float>( testValues, Allocator.TempJob );
JobTest testJob = new JobTest();
testJob.deltaTime = Time.deltaTime;
testJob.result = results;
JobHandle handle = testJob.Schedule( results.Length, 32 );
handle.Complete();
results.CopyTo( testValues );
results.Dispose();
Debug.LogWarning( "0 Index : " + testValues[0] );
}
}
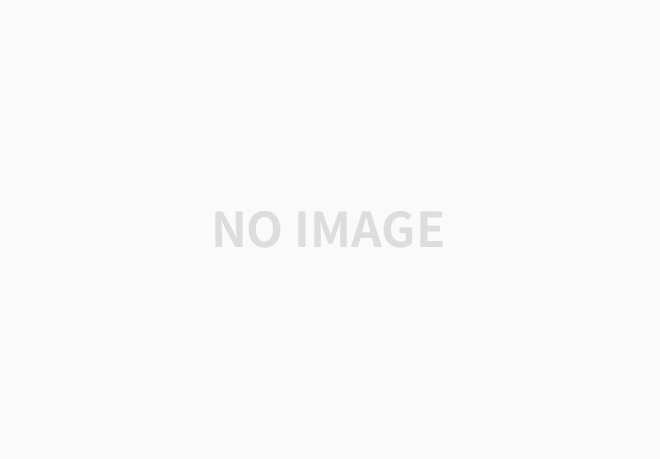
Update() + Job System을 이용했을 때 33 fps 정도의 성능을 보인다.
[BurstCompile( CompileSynchronously = true )]
public struct JobTest : IJobParallelFor
{
public float deltaTime;
public NativeArray<float> result;
public void Execute(int index)
{
result[index] += math.sin( deltaTime );
}
}
public class JobMain : MonoBehaviour
{
int count = 1000000;
float[] testValues;
private void Start()
{
testValues = new float[count];
for( int i = 0; i < count; i++ )
{
testValues[i] = 0;
}
}
void Update()
{
NativeArray<float> results = new NativeArray<float>( testValues, Allocator.TempJob );
JobTest testJob = new JobTest();
testJob.deltaTime = Time.deltaTime;
testJob.result = results;
JobHandle handle = testJob.Schedule( results.Length, 32 );
handle.Complete();
results.CopyTo( testValues );
results.Dispose();
Debug.LogWarning( "0 Index : " + testValues[0] );
}
}
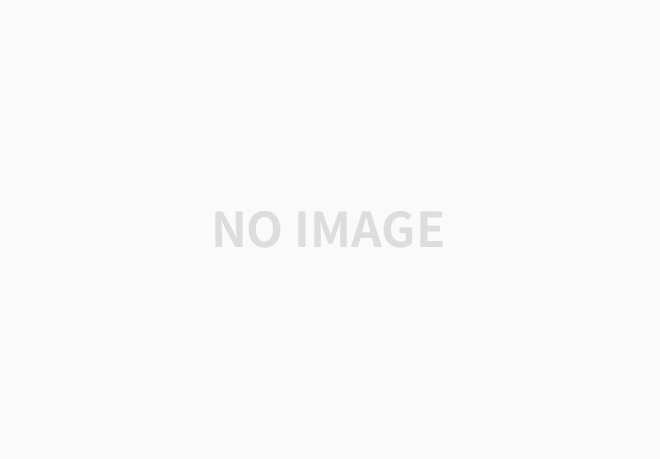
Update() + Job System + Burst을 이용했을 때 216 fps 정도의 성능을 보인다.
Update() 만을 사용했을 때에 비해 Job+Burst를 사용하면 약 18배의 성능 향상이 되는 것을 확인 할 수 있다. 이 때문에 ITween을 대신하여 Job+Burst로 Tween을 구현하려는 것이다.
'DotsTween' 카테고리의 다른 글
[Unity] Job 시스템 이해, JobHandle - (3) (0) | 2020.07.15 |
---|---|
[Unity] Job 시스템 이해, NativeContainer - (2) (1) | 2020.07.15 |
[Unity] Job 시스템 이해, IJob - (1) (0) | 2020.07.15 |
[Unity] Unity Easing 공식 (0) | 2020.07.12 |
[Unity] DotsTween이란? (0) | 2020.07.12 |